Spring Boot — Override config property value before the application startup
In this article we are going to create a spring boot application in which we will set the database password by reading them from the windows credential manager.
Lets create a new spring boot project from https://start.spring.io/ with dependencies Spring web, Spring data jpa, Lombok and MySql driver.

Your project structure should look like this

Open this project in Intellij idea.
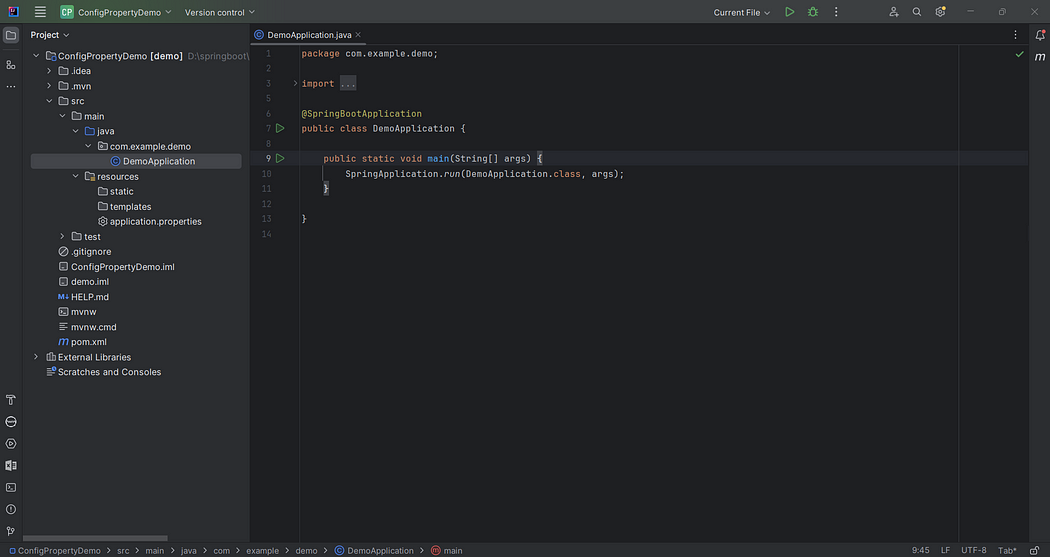
Database
Lets create a mysql database or you can use any existing database. I have created a database by name testdb and we will try to make connection to this database through our spring boot app.

Windows Credential Manager
Lets add an entry in the windows credential manager for our database username and password.

Click on add a generic credential.

Password I used here is root.
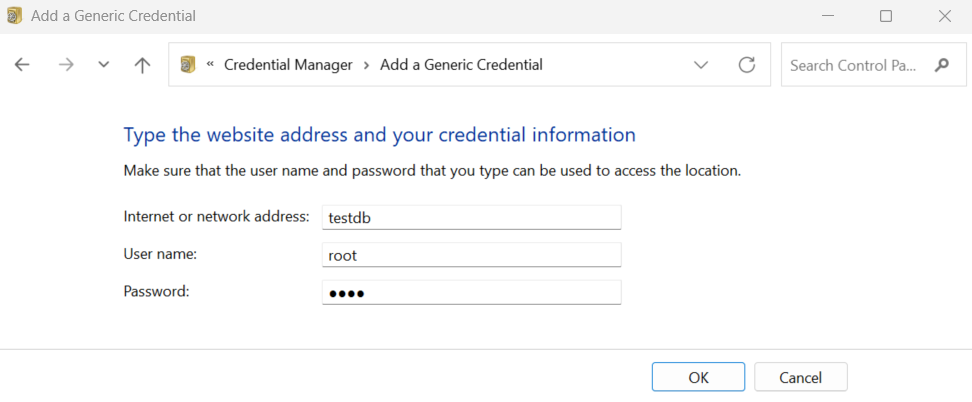
Click OK to save the credential.
Windows Credential Manager Java API
We will be using this Java api to retrieve the username and password from windows credential manager.
You can download the JAR and its dependencies here.
Lets add these JARs to our project.
Create a libs folder inside our project’s root folder and these JARs to that folder.

add following dependencies in the pom.xml file.
<dependency>
<groupId>szczepaniak.dariusz.java.jna</groupId>
<artifactId>windows.credential.manager</artifactId>
<version>0.0.1-SNAPSHOT</version>
<scope>system</scope>
<systemPath>${basedir}/libs/windows.credential.manager-0.0.1-SNAPSHOT.jar</systemPath>
</dependency>
<dependency>
<groupId>net.java.dev.jna</groupId>
<artifactId>jna</artifactId>
<version>5.9.0</version>
<scope>system</scope>
<systemPath>${basedir}/libs/jna-5.9.0.jar</systemPath>
</dependency>
<dependency>
<groupId>net.java.dev.jna</groupId>
<artifactId>jna-platform</artifactId>
<version>5.9.0</version>
<scope>system</scope>
<systemPath>${basedir}/libs/jna-platform-5.9.0.jar</systemPath>
</dependency>
Lets add these database properties in the application.properties file.
db.username=root
db.password=
spring.datasource.url=jdbc:mysql://localhost:3306/testdb
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
spring.datasource.username=${db.username}
spring.datasource.password=${db.password}

we have set the db.password to empty string. Lets run the application.

Application is unable to start and it is showing in the log that we have not provided the password.
Setting the database password
Add a package name config and create a class named ConfigPropertyListener inside it.

/*
com.example.demo.config.ConfigPropertyListener.java
*/
package com.example.demo.config;
import learning.jna.credenumerate.GenericWindowsCredentials;
import learning.jna.credenumerate.WindowsCredentialManager;
import org.springframework.boot.context.event.ApplicationEnvironmentPreparedEvent;
import org.springframework.context.ApplicationListener;
import org.springframework.core.env.ConfigurableEnvironment;
import org.springframework.core.env.PropertiesPropertySource;
import java.util.Properties;
public class ConfigPropertyListener implements ApplicationListener<ApplicationEnvironmentPreparedEvent> {
@Override
public void onApplicationEvent(ApplicationEnvironmentPreparedEvent event) {
ConfigurableEnvironment environment = event.getEnvironment();
WindowsCredentialManager wcm = new WindowsCredentialManager();
GenericWindowsCredentials gwc = wcm.getByTargetName("testdb");
String dbPassword = gwc.getPassword();
Properties properties = new Properties();
properties.put("db.password", dbPassword);
environment.getPropertySources().addFirst(new PropertiesPropertySource("myProperties", properties));
}
}
Code is self-explanatory, we are creating a WindowsCredentialManager object and retrieving GenericWindowsCredentials object along with credential entry we have added to store our database password.
Setting the retrieved password into a properties object and passing the properties object to the method of environment object.
Now create a folder named META-INF inside resources folder and create a file spring.factories inside it, put the following line in that file.
org.springframework.context.ApplicationListener=com.example.demo.config.ConfigPropertyListener
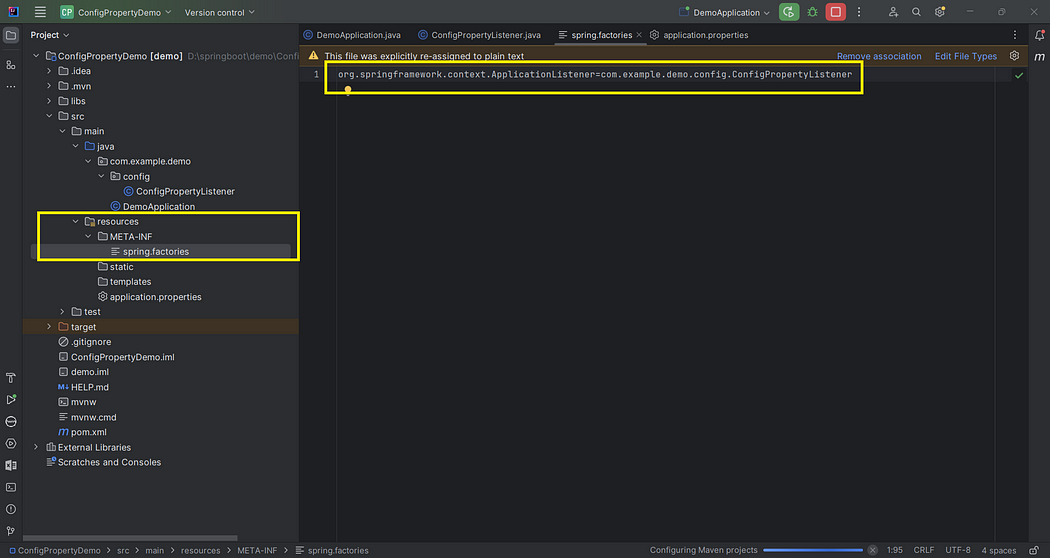
Lets run the application again.

Application started successfully, this means application is able to get the database password from windows credential manager and made a successfull connection to mysql database.
Comments
Post a Comment